Hooks
You can use Python (and Javascript) hook files to do some custom scripting.
In order to find the hook files the file name must match exactly. Please see list below.
The example scripts can be found here:
https://github.com/das-element/resources/tree/main/scripts/hooks/examples
Script | Description |
---|---|
| Gets executed before each transcoding task |
| Gets executed after each transcoding task |
| Gets executed before the file paths get loaded into the ingest view |
| Gets executed before and elements is delete from the library |
| Gets executed after and elements got delete from the library |
| Gets executed before the library elements get exported from the Settings → Library → This is not drag&drop of elements into another application! Use the drag&drop notations or |
| Gets executed right after the drag of an element. Useful to customize and format the dragged data outside of the software. Please note that this is a Javascript file. |
The software follows this order looking for Python Hook files.
directory defined in the
$DASELEMENT_HOOKS
environment variabledirectory defined in the
$DASELEMENT_RESOURCES/scripts/hooks
environment variableyour local
.das-element
folder (for Linux:~/.das-element/scripts/hooks
or for Windows%homepath%/.das-element/scripts/hooks
)
The result data needs to be JSON serializable.
For example convert pathlib Path to string → str(Path('/some/path/file.exr'))
Pre Render Hook
The input is the resolver data dictionary
which will be used to resolve the path pattern. You can modify it here before the transcoding task gets processed.
You will need to return the same dictionary with your changes included.
In the example below, in order to resolve a path pattern like <custom.dependecy>
you need to add some value for the custom data. If that is not provided the resolve would otherwise fail if you re-render the proxies and there is no main task to provide the dependency which we normally would get from the post render hook after sending the task to the render farm.
Link to example script:
https://github.com/das-element/resources/blob/main/scripts/hooks/examples/deadline/pre_render.py
Post Render Hook
The input is the output of the process called by the custom command task.
You will have to return a Dictionary which will be added as custom
to the resolver data and can later be accessed in the Path Builder. return {'dependency': job_id}
can later be resolved with <custom.dependency>
Link to example script:
https://github.com/das-element/resources/blob/main/scripts/hooks/examples/deadline/post_render.py
Pre Ingest Load
This hook can be used to parse the file paths to set tags and the category from the file path before the items are loaded into the ingest list. These tags and the category will automatically populate the ingest list items.
The input is a List of Dictionaries and the same has to be returned from the function.
Link to example script:
https://github.com/das-element/resources/blob/main/scripts/hooks/examples/ingest/pre_ingest_load.py
Pre Export
This hook can be used to edit, reformat or add additional values to the export elements from the settings page of the library.
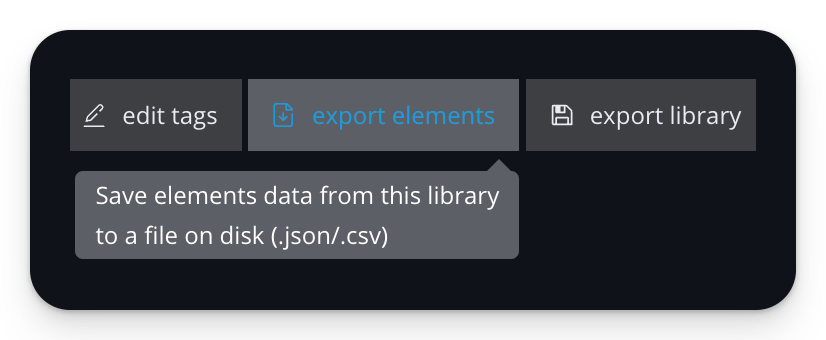
Tip: use this hook to create a CSV file that you can import into Shotgrid
The input is a List of Dictionaries and the same has to be returned from the function.
Link to example script:
https://github.com/das-element/resources/blob/main/scripts/hooks/examples/shotgrid/pre_export.py
Pre / Post Element Delete
Hook file that gets exectued right befor and after an element was delete from the library and database. Allows custom cleanup and deletion of files.
By default software will only delete path files defined in the database:
path
path_thumbnail
path_filmstrip
path_proxy
Pre Gallery Drag
Use this hook to customize and format the data that is being dragged outside of the software.
It will drag a String format of the data that you specified in the hook file.
Please note that this is a Javascript file.
Troubleshooting
Logger inside python file
Since version 2.1.2
you can directly access the logger inside the hook files.
def main(*args, logger=none):
items = args[0]
# use the Das Element logger
# will log to ~/.das-element/logs/
logger.warning('This is a warning!')
return items
if __name__ == '__main__':
main(sys.argv[1:])
Example for pre_load_ingest.py
import logging
def main(*args, logger=none):
items = args[0]
logger.warning('This is a warning!') # <- use the Das Element logger (~/.das-element/logs/)
logging.warning('This is a warning!') # <- use the default logging module
for item in items:
print(item) # <- this print() will be output to the console
return items
if __name__ == '__main__':
main(sys.argv[1:])